Kexi/Junior Jobs/Add d-pointers: Difference between revisions
< Kexi | Junior Jobs
Line 11: | Line 11: | ||
Classes that have to be converted are in this form: | Classes that have to be converted are in this form: | ||
<source lang="cpp-qt"> | |||
// MyClass.h | // MyClass.h | ||
class MyClass { | class MyClass { | ||
Line 23: | Line 24: | ||
m_foo/doSomething(); | m_foo/doSomething(); | ||
} | } | ||
</source> | |||
After converting to d-pointer it should be: | After converting to d-pointer it should be: | ||
<source lang="cpp-qt"> | |||
// MyClass.h | // MyClass.h | ||
class MyClass { | class MyClass { | ||
Line 52: | Line 55: | ||
delete d; | delete d; | ||
} | } | ||
</source> | |||
==Extra tasks needed== | ==Extra tasks needed== |
Revision as of 19:53, 21 November 2011
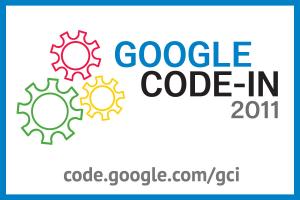
- OPEN, DIFFICULTY=2/5
- Recommended for Google Code-IN program
Goal
Improve internal APIs in Kexi. This can be done easily by introducing d-poitners to classes that lack them.
Introduction
Read section about d-pointers on Techbase (d-pointers only, not shared d-pointers).
Classes that have to be converted are in this form:
// MyClass.h
class MyClass {
public:
MyClass();
private:
Foo m_foo;
};
// MyClass.cpp
MyClass::MyClass() {
m_foo/doSomething();
}
After converting to d-pointer it should be:
// MyClass.h
class MyClass {
public:
MyClass();
private:
class Private;
Private * const d;
};
// MyClass.cpp
class MyClass::Private {
public:
Private()
Foo foo;
};
MyClass::MyClass()
: d(new Private)
{
d->foo.doSomething();
}
MyClass::~MyClass()
{
delete d;
}
Extra tasks needed
After converting to d-pointer:
- any code referring to m_foo attribute should be changed to d->foo
- if there is inline code in header file that uses member variable, do not move the variable to d-pointer unless you know for sure it isn't there for optimization
Required skills
Basic Qt, average C++.
- Work within a new branch kexi-dpointers-gci, please create.
Notes to mentor
- Please always emphasize importance of coding style to students and check that - it's so easier to maintain the style from day one instead of fixing afterwards. Show Techbase articles for that.
- Take care of coding practices too (see Techbase articles)